Retrieve data from multiple tables using JOINs in MySQL
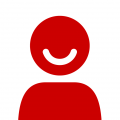
Hello, all!
This is my first post after just joining this discussion, so please forgive me and provide kind assistance if I have posted to the wrong subsection!
How can I retrieve data from multiple tables using JOINs in MySQL to create a comprehensive report of customer orders including their names, order dates, and product details?
This is my first post after just joining this discussion, so please forgive me and provide kind assistance if I have posted to the wrong subsection!
How can I retrieve data from multiple tables using JOINs in MySQL to create a comprehensive report of customer orders including their names, order dates, and product details?
Thankyou in advance.
Answers
You can use the SELECT statement with JOIN clauses. Assuming you have tables for customers, orders, and products, here's an example query:
With this query, you use INNER JOINs to connect these tables based on their relationships (customer_id, order_id, product_id). Adjust the column names and table names according to your actual database schema.
This query retrieves information about each customer, their orders, and the products in those orders. You can customize the SELECT clause to include additional columns or modify the WHERE clause to filter the results based on specific conditions.
Note:
If there are customers who haven't placed orders or orders without associated details, you might want to use LEFT JOINs to include all customers or orders in the result set, even if there are no corresponding records in the joined tables. Adjust the JOIN type based on your data and reporting requirements.