How do i know my dll is obfuscated with a non trial version
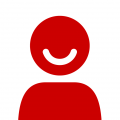
I need my application to tell me if it has been obfuscated with SmartAssembly trial or professional. I need it to open a messagebox that says if the version I used is trial and therefore expires or if I used the professional. How do you do this in C #? What code should I write? What dll should I use?
Tagged:
Answers
The best way would be to look for custom attributes added to the assembly by SA. Each assembly processed by SA will have PoweredByAttribute on it and those processed with trial version, will additionally have DoNotDistributeAttribute on them.
So, you can use System.Reflection or something similar and look if there is:
I imagine you are wanting to check this before sending the application out - but otherwise if you try to launch application processed with trial version, on machine that doesn't have the SA installed (or expiration date was reached), it'll show a messagebox and then quit.
Victoria Wiseman | Redgate Software
Have you visited our Help Center?
This should work, the trial version expires and turns into the free version. You should still be able to do testing with the free version and you don't need to worry about the trial keys.
Victoria Wiseman | Redgate Software
Have you visited our Help Center?
My point is I required two trial licenses as my development and testing environments are different. Red-Gate provides only single trial license per Company. However I have been offered second trial license from Red-Gate team.
Thanks.