Progress feedback
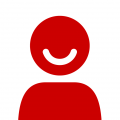
I am working with sdk 7 and it works like a charm. I am building some .Net components that will snapshot various database and running them as a windows service.
I am also creating a console utility to do the same and would like to provide feedback to the console. Is there a way to call CompareDatabases async and update the console on progress, i.e., which table is being compared as in the Redgate windows apps.
I am also creating a console utility to do the same and would like to provide feedback to the console. Is there a way to call CompareDatabases async and update the console on progress, i.e., which table is being compared as in the Redgate windows apps.
Comments
Thanks for your post! You can update the console window by assigning a Status event to the Session object and then running the CompareDatabases method in a new thread. I hope that you can use the following sample code: