Exception not found, although thrown
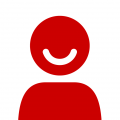
I have a particular piece of code where EH1 just reports one possible exception (System.ArgumentException) but it definitely throws a System.IndexOutOfRangeException
Here is the code in question:
The two functions SegmentSum() and SegmentClear() do not throw any exception. _bandwidth is a settings container.
Any clues?
Here is the code in question:
private const int _maxSegments = 10; private Object SyncRoot = new Object(); private Bandwidth _bandwidth; private int[] _segments = new int[_maxSegments]; private int _lastSegment; private long _maxBytesPerSegment; private long _currentlyUsedBytes; public void CountAndWait(int nBytes) { lock(SyncRoot) { int nTickCount = Environment.TickCount; int nTickSegment = (nTickCount / (1000 / _maxSegments)) % _maxSegments; _currentlyUsedBytes = SegmentSum(); long maxBytes = _bandwidth.MaxBytesPerSecond; if(maxBytes == 0 || _bandwidth.IsUnlimited) { return; } if(maxBytes < nBytes) { SegmentsClear(); System.Threading.Thread.Sleep(1000); return; } if(_segments[nTickSegment] + nBytes > _maxBytesPerSegment) { System.Threading.Thread.Sleep(1000 / _maxSegments); nTickSegment = (nTickSegment + 1) % _maxSegments; _segments[nTickSegment] = nBytes; } else { _segments[nTickSegment] += nBytes; } int nOldestTickSegment = (nTickSegment + 1) % _maxSegments; if(_lastSegment != nTickSegment) { _segments[nOldestTickSegment] = 0; _lastSegment = nTickSegment; } } }
The two functions SegmentSum() and SegmentClear() do not throw any exception. _bandwidth is a settings container.
Any clues?
Comments
The best thing I can suggest is to turn on or off the use more detailed analysis setting. Exception Hunter tries to eliminate exceptions that cannot possibly happen based on conditional branches and the like. For instance if you test for a null and skip a line of code that can throw a null-reference exception based on the findings, Exception Hunter can work out that the null-reference can never logically happen.
I think you're encountering a limitation of the algorithm used by Exception Hunter: it does not report all possible exceptions generated by the .NET runtime. Usually when we can't determine with certainty whether or not an exception will be thrown we err on the side of caution and report it.
However, the IndexOutOfRangeException thrown by the runtime on some array accesses is an exception to this general rule. The problem is that our model doesn't extend to array sizes and has only limited support for integer values: not enough to ever be able to determine whether or not an array access is safe or not.
Array accesses are so common in the .NET framework that this means that we would report this exception for nearly every non-trivial function. The overwhelming majority of these would be from safe code that never throws the exception in reality. We decided that as this exception would be reported everywhere it would be better not to report it as it would be rare that the information is actually useful.
I think that there are improvements that may be made so that we could detect some more obvious cases where this could happen, though there would be concerns about the effect this would have on the performance of the tool.
Software Developer
Red Gate Software Ltd.
Already tried various settings to see if EH1 shows more info. Without success in this case.
Well, time to engage other tricks.