Recognizing handled exceptions
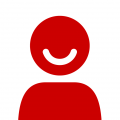
I ran ExceptionHunter on some test code to see what it would do. When i was provided with the possible exceptions, I added and if statement that threw the correct exceptions the condition was met, however when I run ExcpetionHunter again, it still shows me that the exception condition exists.
Here is my code
No exception handling
public bool StringParameterMethod( string value )
{
if ( value.Equals( "ABC" ) )
{
return true;
}
return false;
}
With exception handling
public bool StringParameterMethod_Valid(string value)
{
if (value == null) { throw new NullReferenceException("The provided parameter {value} was null"); }
if (value.Length == 0) { throw new ArgumentOutOfRangeException("Value", value, "The provided parameter {value} did not contain a valid value." ); }
if (value.Equals("ABC"))
{
return true;
}
return false;
}
Am I doing something wrong?
Here is my code
No exception handling
public bool StringParameterMethod( string value )
{
if ( value.Equals( "ABC" ) )
{
return true;
}
return false;
}
With exception handling
public bool StringParameterMethod_Valid(string value)
{
if (value == null) { throw new NullReferenceException("The provided parameter {value} was null"); }
if (value.Length == 0) { throw new ArgumentOutOfRangeException("Value", value, "The provided parameter {value} did not contain a valid value." ); }
if (value.Equals("ABC"))
{
return true;
}
return false;
}
Am I doing something wrong?
Derik Whittaker
http://devlicio.us/
http://devlicio.us/
Comments
With the following code Exception Hunter will tell you you throw all of the exceptions for Equals as well as NullReferenceException/ArgumentOutOfRangeException (Unless it can determine that any of them will never be thrown).
public bool StringParameterMethod_Valid(string value)
{
if (value == null) { throw new NullReferenceException("The provided parameter {value} was null"); }
if (value.Length == 0) { throw new ArgumentOutOfRangeException("Value", value, "The provided parameter {value} did not contain a valid value." ); }
if (value.Equals("ABC"))
{
return true;
}
return false;
}
To stop exceptions you would need to add a try...catch handlers to do somthing when the exceptions are thrown, generally you might want to do this in the functions which call StringParameterMethod_Valid or you might want to wrap your call to Equals with a try...catch and return false, IE:
public bool StringParameterMethod_Valid(string value)
{
try
{
if (value.Equals("ABC"))
{
return true;
}
} catch (ArgumentOutOfRangeException argumentOutOfRangeException)
{
// Log if needed
return false; // not valid
}
catch(NullReferenceException nullReferenceException)
{
//Log as needed
return false; // not valid
}
return false;
}
HTH,
James
Head of DBA Tools
Red Gate Software Ltd
I understand what you are saying, I just don't agree with it. I should NOT have to put a try-catch in order for ExceptionHunter to think I have handled the possible exception case.
From my point of view, this violates the fail-fast concept. If i only have a try-catch, then I am not doing my part in checking for possible known failures before processing. I understand that I need try-catches, but try-catches should be for unknown (at development time) exceptions.
I really believe the product should notice the fact that the exception scenario has been handled show you that.
http://devlicio.us/
When I run analysis on the following code I get told a nullreferenceexception can be thrown:
private static bool MyFunction(string p)
{
if (p.Equals("ABC"))
{
return true;
}
return false;
}
If I add
if (p == null)
{
return false;
}
Before the p.Equals I get told that no exceptions can be thrown.
With your code I would expect to be told a NullReferenceException or ArgumentOutOfRangeException can be thrown as you are throwning them explicitly (their source will be different from your original example without error handling but they are still thrown).
I am not quite sure what output you are expecting from your second example.
James
Head of DBA Tools
Red Gate Software Ltd
private static string GetDateString(DatePicker date)
{
try
{
if (date != null && date.SelectedDate != null)
{
return date.SelectedDate.Value.ToString("yyyy/MM/dd");
}
return DateTime.MinValue.ToString("yyyy/MM/dd");
}
catch (NullReferenceException)
{
return DateTime.MinValue.ToString("yyyy/MM/dd");
}
}
I get an error that System.Windows.Controls.DataPicker.get_SelectedDate() may raise a NullReferenceExcpetion
I have clearly dealt with it twice, I don't see why exception hunter is still showing it as an unhandled exception.