profiling XPS
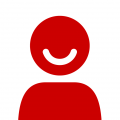
Hello,
I'm trying to profile a little test-application with our registered ANTS Profiler v2.7.1.20 and I always get the message "The application you're profiling has exited without shutting down normally. Profiling will now stop." even though the application do not throw an exception (at least I don't see any!)... btw, I have also tried with latest version 3.1 (trial)
I get this message when using the SerializerWriterCollator class in the test-application, without it works fine...
what's going wrong here? Is there a bug in the MS assembly or is it an issue of the ANTS profiler?
if it's a bug in the MS assembly I will inform Daniel Emmerson of Microsoft about... (c;
Any help would be great...
kind regards from Germany
Jo
here's the little sample code I have used:
I'm trying to profile a little test-application with our registered ANTS Profiler v2.7.1.20 and I always get the message "The application you're profiling has exited without shutting down normally. Profiling will now stop." even though the application do not throw an exception (at least I don't see any!)... btw, I have also tried with latest version 3.1 (trial)
I get this message when using the SerializerWriterCollator class in the test-application, without it works fine...
what's going wrong here? Is there a bug in the MS assembly or is it an issue of the ANTS profiler?
if it's a bug in the MS assembly I will inform Daniel Emmerson of Microsoft about... (c;
Any help would be great...
kind regards from Germany
Jo
here's the little sample code I have used:
using System; using System.Collections.Generic; using System.Text; using System.IO; using System.Diagnostics; using System.Windows.Xps.Packaging; using System.Windows.Media; using System.Windows.Documents.Serialization; using System.Windows; namespace XpsTest { class Program { static void Main(string[] args) { try { // create a test xps-file with 1 page using visuals using (XpsDocument xpsDocument = new XpsDocument("test.xps", System.IO.FileAccess.Write)) { XpsDocumentWriter xpsDocumentWriter = XpsDocument.CreateXpsDocumentWriter(xpsDocument); SerializerWriterCollator writerCollator = xpsDocumentWriter.CreateVisualsCollator(); writerCollator.BeginBatchWrite(); writerCollator.Write(CreateVisual(793.76, 1122.56, "Index 0815/4711")); writerCollator.EndBatchWrite(); } Console.WriteLine("test done."); Console.ReadLine(); } catch (Exception ex) { Console.WriteLine("Exception: {0}", ex.Message); } } public static Visual CreateVisual(double width, double height, string message) { // create a visual DrawingVisual visual = new DrawingVisual(); using (DrawingContext ctx = visual.RenderOpen()) { // draw some text... FormattedText text = new FormattedText(message, System.Globalization.CultureInfo.CurrentCulture, System.Windows.FlowDirection.LeftToRight, new Typeface("Arial"), 10 * 1.5, new SolidColorBrush(Color.FromRgb(0, 0, 0)) ); ctx.DrawText(text, new System.Windows.Point(100, height - 125)); // ..and drawe some lines Pen pen = new Pen(new SolidColorBrush(Color.FromRgb(0, 0, 0)), 1); ctx.DrawLine(pen, new Point(20, 100), new Point(30, 100)); ctx.DrawLine(pen, new Point(20, 110), new Point(30, 110)); ctx.DrawLine(pen, new Point(20, 120), new Point(30, 120)); ctx.DrawLine(pen, new Point(20, 130), new Point(30, 130)); ctx.DrawLine(pen, new Point(20, 140), new Point(30, 140)); ctx.DrawLine(pen, new Point(20, 150), new Point(30, 150)); ctx.DrawLine(pen, new Point(20, 160), new Point(30, 160)); ctx.DrawLine(pen, new Point(20, 170), new Point(30, 170)); ctx.DrawLine(pen, new Point(20, 180), new Point(30, 180)); ctx.DrawLine(pen, new Point(20, 190), new Point(30, 190)); ctx.DrawLine(pen, new Point(20, 200), new Point(30, 200)); ctx.DrawLine(pen, new Point(20, 210), new Point(30, 210)); ctx.DrawLine(pen, new Point(20, 220), new Point(30, 220)); ctx.DrawLine(pen, new Point(20, 230), new Point(30, 230)); ctx.DrawLine(pen, new Point(20, 240), new Point(30, 240)); } return visual; } } }
Comments
It's probably not a bug in the MS components, to be honest, because this error normally indicates a failure to instrument the Intermediate Language code. I have an internal fix for the core2.dll component of ANTS Profiler that fixes this problem in one particular circumstance and it may work for you as well. You will need to use the 3.1 version (demo) if you want to apply it.
I have a 32-bit and a 64-bit version of the fix, so I would need to know which version you require. How can I get the dll to you? Can I email it?
thanx for your quick reply and info about this issue, much appreciated and ofcoz I'd like to give it a try, even if it's only for the 3.1 (trial) version.
Can you try to send the dll (actually 32bit would be fine, but you could also send both version) to the email-address which I've used to register here?
However just let me know if it doesn't work or you'll need an offical email/ftp-account or similar (license number etc), then I'll PM you the infos on monday when I'm back at work...
once again, thanx for your quick reply and help,
kind regards from Germany
Jo
today we have ugraded our license for ANTS Profiler from version 2.7.1 to 3.1 ... is that fix already applied to that version or do we still need the dll's to fix this issue? I guess so...
can you send'em via email?
regards,
Jo
I don't have anything I can apply to v2.71 of the ANTS Profiler core; just the 3.1 version. And no guarantees as I don't know for sure if it's the same problem. Does it work in an unpatched 3.1? Then I wouldn't get the fix from me because it may well cause more problems.
I have installed and registered the 3.1 version now and it shows same behaviour, like I've already mentioned in my initial first post...
So it would be nice to try out that fix to see if it helps on that issue, else we are lost in profiling using ANTS profiler,
as it seems it has problems with all those applications heavy using xps-classes and others from .NET 3.0 framework (like WCF/WPF etc.) ...
regards,
Jo
I'll email the dll to the email address that you have registered on the forums with. I don't think the problem is specific to .NET 3.0/3.5, but down to the programming practices of the person who wrote that code. There is a particular situation with the way exceptions are thrown and that's the basic cause of the problem.
The fix is on the way!
thanx for the information about the issue and sending the dll.
I have downloaded it and replaced the original RedGate.Profiler.Core2.dll with the one you've sent me, and rebootet system. Do I need to register the dll or similar?
Seems like the fix doesn't help - we still can't profile some of our applications, not even the simple test-program I've posted above in my initial first post...
hm...and now?
regards,
Jo
It looks like the code gets stuck because of some finalization issue when it's being profiled. When the application tries to exit, a finalizer cannot run, then .NET 2's critical finalizer kicks in after 40 seconds and tries to force the object out of memory and fails, so the thread dies. I think the stubborn object is an MS.Internal.FontCache.LPCMessage (whatever that is). I got this information by trying to take snapshots after pressing return in the sample application before it crashes and examining the thread with the highest ID (the critical finalizer). Apparently these LPC Messages and ANTS Profiler's core don't get along. I'll try to find out why.
thanx for investigating this issue and explanation about the problem. Those LCP messages might be messages sent to the "PresentationFontCache.exe", a lokal service for the xps/wpf caching fonts etc... but this is just a wild guess...
I hope you'll find out more, would be really nice if we could also use that mighty (and imho probably the best profiler!) ANTS profiler for the rest of our applications we need to profile/meassure...
drop me a note if by any chance I can help or you'll need more informations... I could also help on testing a new fix (if there's one) with our applications having problems with...
regards,
Jo
I'm not sure this can be fixed, so I will log a bug to the developers to see if there is perhaps a clever workaround. The problem seems to stem from the fact that, internally, the AppDomain is relying on .NET 2.0's critical finalizer to clean up the FontCache object's unmanaged resources. When the critical finalizer runs, it attempts to free all resources used by your application, which includes the ANTS Profiler named pipe as well.
The named pipe is used to transfer method data from your application to Profiler's front-end. When you get past the last line of code, the critical finalizer kicks in and closes the ANTS Profiler named pipe, causing the UI to terminate profiling. You can see this if you enable logging in Profiler (create a logs subfolder) where you will see the pipe being closed: This does not totally prevent you from using ANTS Profiler, though, as you can still take snapshots rather than relying on ANTS Profiler to send the data automatically when the application is closed. Even if you want to see what is happening in the critical finalizer, you still have a good 40 seconds to take a snapshot after you press RETURN in your console window.
While we will endeavour to fix this, it does seem to be a special case brought about how WPF handles unmanaged resources internally.
thanx for logging this issue as a bug to the developers... let's see if they'll find a work-around or a fix for that problem.
Meanwhile I'll try to profile using the snapshot, though this is not always possible or hard to do, depending on the application and their tasks...
Next week I'm off for 4 days vacation, so "no hurry" about, but I'll keep crossing fingers for the developers... (c;
thanx for all help so far,
kind regards from Germany
Jo
any news to this issue? were the developers able to find a work-around or fix for that problem?
best wishes from Germany,
Jo
I'm afraid not. There isn't anything we can do about another thread closing our pipe, so preventing the critical finalizer would be the only way out, and I'm not sure that can be done.
that aren't good news...
Does that mean all applications using XPS (or WPF) will have problems on profiling?? hm...
regards from Germany,
Jo
It's probably going to happen in only rare occurrances. It doesn't mean that you can't use WPF or wahtever. Neither does it prevent you from using the profiler. You just can't rely on getting results when you close the application; you have to use 'take snapshot' instead to get your results.