(info) GetFileStream method of the ExecutionBlock Object
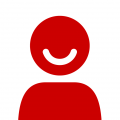
Hi all,
There is a bit in the Toolkit help file that mentions using the
GetFileStream method to save a migration script to a file and I thought I'd
explain a bit about what it does.
If you produce a migration script ExecutionBlock using the Toolkit using
GetMigrationSql (in DataCompare.Engine) or BuildFromDifferences (in
SQLCompare.Engine), you can simply get the SQL script using the ToString()
method on the ExecutionBlock. That works just fine, but it's inefficient. On
larger scripts, there is the potential that the string could be cut off at
the end or worse because of the way the ToString method works. Apparently,
it uses more system resources because it needs to allocate memory for the
entire output string as well as its' work buffers, so on large SQL scripts
there is a potential burden on your system resources.
You can save these resources by using the GetFileStream method, which
returns a read file handle on the migration SQL, effectively allowing you to
read small 'chunks' of the SQL into a buffer so they can be written directly
to disk. This will save a tremendous amount of system memory if you have
produced gigabytes of migration SQL!
Here is a C# example function that you can feed an execution
block and an output file name to as arguments that will write the execution
block to a file:
static void SaveMigrationScript(ExecutionBlock eb, string OutFileName)
{
/* get filestream from execution block */
FileStream InFS = eb.GetFileStream();
/* Open an output file named OutFileName */
FileStream OutFS = new FileStream(OutFileName,
System.IO.FileMode.Create);
int ByteCount = 0; /* Count number of bytes read from the
execution block */
int BytesTotal = (int) InFS.Length; /* How many bytes to write
total */
int BUFLEN=256; /* The size of our file buffer */
int offset=0; /* Where we are at at the moment */
Console.WriteLine("Writing " +BytesTotal +" bytes to
"+OutFileName);
Byte[] buffer = new Byte[BUFLEN];
while (offset < BytesTotal)
{
/* Read from FileStream */
ByteCount = InFS.Read(buffer, 0, BUFLEN);
offset += ByteCount;
/* Write to output file */
OutFS.Write(buffer, 0, ByteCount);
}
/* Close output file */
OutFS.Close();
}
Note: This article is valid for versions 3.10, 3.15, 3.16, and 3.37 of
SQL Toolkit. No warranty is given or implied on this code, use at your own
discretion.
There is a bit in the Toolkit help file that mentions using the
GetFileStream method to save a migration script to a file and I thought I'd
explain a bit about what it does.
If you produce a migration script ExecutionBlock using the Toolkit using
GetMigrationSql (in DataCompare.Engine) or BuildFromDifferences (in
SQLCompare.Engine), you can simply get the SQL script using the ToString()
method on the ExecutionBlock. That works just fine, but it's inefficient. On
larger scripts, there is the potential that the string could be cut off at
the end or worse because of the way the ToString method works. Apparently,
it uses more system resources because it needs to allocate memory for the
entire output string as well as its' work buffers, so on large SQL scripts
there is a potential burden on your system resources.
You can save these resources by using the GetFileStream method, which
returns a read file handle on the migration SQL, effectively allowing you to
read small 'chunks' of the SQL into a buffer so they can be written directly
to disk. This will save a tremendous amount of system memory if you have
produced gigabytes of migration SQL!
Here is a C# example function that you can feed an execution
block and an output file name to as arguments that will write the execution
block to a file:
static void SaveMigrationScript(ExecutionBlock eb, string OutFileName)
{
/* get filestream from execution block */
FileStream InFS = eb.GetFileStream();
/* Open an output file named OutFileName */
FileStream OutFS = new FileStream(OutFileName,
System.IO.FileMode.Create);
int ByteCount = 0; /* Count number of bytes read from the
execution block */
int BytesTotal = (int) InFS.Length; /* How many bytes to write
total */
int BUFLEN=256; /* The size of our file buffer */
int offset=0; /* Where we are at at the moment */
Console.WriteLine("Writing " +BytesTotal +" bytes to
"+OutFileName);
Byte[] buffer = new Byte[BUFLEN];
while (offset < BytesTotal)
{
/* Read from FileStream */
ByteCount = InFS.Read(buffer, 0, BUFLEN);
offset += ByteCount;
/* Write to output file */
OutFS.Write(buffer, 0, ByteCount);
}
/* Close output file */
OutFS.Close();
}
Note: This article is valid for versions 3.10, 3.15, 3.16, and 3.37 of
SQL Toolkit. No warranty is given or implied on this code, use at your own
discretion.
This discussion has been closed.