Generate Random Date in Python
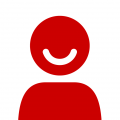
Hi,
I'm having real trouble using Python Script to generate a random date between two dates. Ideally I'd like to call something like the following:
GenerateDate(DateOfBirth,"01/03/2014")
and for that to return be a random date between the DateOfBirth column and 1st March 2014.
Does anyone have any examples of this?
Many thanks,
Andrew
I'm having real trouble using Python Script to generate a random date between two dates. Ideally I'd like to call something like the following:
GenerateDate(DateOfBirth,"01/03/2014")
and for that to return be a random date between the DateOfBirth column and 1st March 2014.
Does anyone have any examples of this?
Many thanks,
Andrew
Comments
Thanks for this. Just what I needed. Although I am having an issue when generating at scale. I have created a SQL table with two datetime columns, DateOfBirth and DateOfEvent. I am generating a random date into DateOfBirth using the standard SQL datetime generator and then I have implemented your script to generate values for DateOfEvent.
This works fine when generating 1,000 records but when generating 1,000,000 records I get the generation error below:
[dbo].[zzRedGateTest]
The value '' cannot be inserted into column DateOfEvent
RedGate.SQLDataGenerator.Engine.DataGeneration.InvalidColumnDataException: The value '' cannot be inserted
into column DateOfEvent at œœœœ.œœœœ.œœœœ() at œœœœ.œœœœ.Read() at
RedGate.SQLDataGenerator.Engine.DataGeneration.TypeTranslationDataReader.Read() at
System.Data.SqlClient.SqlBulkCopy.ReadFromRowSource() at
System.Data.SqlClient.SqlBulkCopy.WriteToServerInternal() at
System.Data.SqlClient.SqlBulkCopy.WriteRowSourceToServer(Int32 columnCount) at
System.Data.SqlClient.SqlBulkCopy.WriteToServer(IDataReader reader) at œœœœ.œœœœ.œœœœ(CancellableController œœœœInt32 œœœœ, ConnectionProperties œœœœ, GenerateAction œœœœ, SqlConnection œœœ, SDGProject œœœœ, GenerationReport Inserted 0 rows
Generation started at 14 March 2014 09:44:48, taken: 00:01:18 (hh:mm:ss)
I can't see how empty strings would be inserted into the value. Could you please let me know how to resolve this issue?
Many thanks,
Andrew
I would have expected some sort of timeout when opening a project if a server cannot be reached, but it just seems to hang forever. As mentioned you have to go into task manager to kill the process, hitting "cancel" doesn't do anything.