Website via FTP
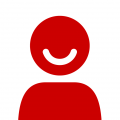
This script deploys a website (or perhaps a folder of any other files) via FTP . It doesn't delete files that are not in the package, as I have virtual directories also in the FTP root.
It's being used for this website: http://www.bluebirdtechnology.com/
My package contains the published website files, and the following deploy.ps1.
I put the web app and videos for the web app in two separate packages with the same script, to reduce the size of the package that changes most frequently.
I hope this helps,
Justin
It's being used for this website: http://www.bluebirdtechnology.com/
My package contains the published website files, and the following deploy.ps1.
I put the web app and videos for the web app in two separate packages with the same script, to reduce the size of the package that changes most frequently.
#Inputs: #$domain = "MyDomain.com" #$ftpPassword = "********" $ftpUrl = "ftp://ftp.$domain/wwwroot" $ftpUserName = $domain Function FtpUpload ([System.Uri]$uri, [string]$username, [string]$password, [string]$filePath) { $ftp = [System.Net.FtpWebRequest]::Create($uri) $ftp = [System.Net.FtpWebRequest]$ftp $ftp.Method = [System.Net.WebRequestMethods+Ftp]::UploadFile $ftp.Credentials = new-object System.Net.NetworkCredential($username,$password) $ftp.UseBinary = $true $ftp.UsePassive = $true $content = [System.IO.File]::ReadAllBytes($filePath) $ftp.ContentLength = $content.Length $rs = $ftp.GetRequestStream() $rs.Write($content, 0, $content.Length) $rs.Close() $rs.Dispose() } Function FtpCreateFolder ([System.Uri]$uri, [string]$username, [string]$password) { $ftp = [System.Net.FtpWebRequest]::Create($uri) $ftp = [System.Net.FtpWebRequest]$ftp $ftp.Method = [System.Net.WebRequestMethods+Ftp]::MakeDirectory $ftp.Credentials = new-object System.Net.NetworkCredential($username,$password) try { $rs = $ftp.GetResponse() } catch [System.Exception] { } if( $rs -ne $null ) { $rs.Close() $rs.Dispose() } } $myDir = Split-Path -Parent $MyInvocation.MyCommand.Path cd $myDir $files = Get-ChildItem -recurse foreach($file in $files) { $directory = $null if ($file.DirectoryName -ne $null) { $directory = $file.DirectoryName.Replace($myDir,"") } $directory += "/"; $uri = New-Object System.Uri($ftpUrl + $directory + $file.Name) if( $file.Extension -eq ".nupkg" ) { continue } # if a folder if( $file.DirectoryName -eq $null) { "Create folder, URI: $uri" FtpCreateFolder $uri $ftpUserName $ftpPassword } else { "Upload file, URI: $uri, File: " + $file.FullName FtpUpload $uri $ftpUserName $ftpPassword $file.FullName } }
I hope this helps,
Justin
Justin Caldicott
Developer
Redgate Software Ltd
Developer
Redgate Software Ltd