Windows application, using Advanced Installer and FTP
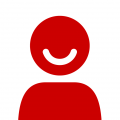
This script deploys an installer to a central location, via FTP, for download via a check for updates mechanism. In this case, I'm using the updates system that is part of the Advanced Installer tool. There's code here to build an updates INI file too, and include release notes from a text file.
It's being used for this Windows app: http://www.bluebirdtechnology.com/
My package has the installer, deploy.ps1 and ReleaseNotes.txt files together in the root.
In the application, I configure the update "channel" to point to the appropriate location on the website, eg. Downloads/Testing/MyApplicationSetup.exe.
Here's the deploy.ps1:
I hope this helps,
Justin
It's being used for this Windows app: http://www.bluebirdtechnology.com/
My package has the installer, deploy.ps1 and ReleaseNotes.txt files together in the root.
In the application, I configure the update "channel" to point to the appropriate location on the website, eg. Downloads/Testing/MyApplicationSetup.exe.
Here's the deploy.ps1:
# Inputs: #$RedGateReleaseNumber = "3.1.353.0" #$RedGatePackageVersion = "3.1.353.0" #$RedGateEnvironmentName = "Testing" $ftpDownloadsUrl = "ftp://ftp.MyDomain.com/wwwroot/Downloads/MyProduct/" + $RedGateEnvironmentName $ftpUserName = "MyUserName" $ftpPassword = "MyPassword" $httpDownloadsUrl = "http://www.MyDomain.com/Downloads/MyProduct/" + $RedGateEnvironmentName Function FtpDelete ([System.Uri]$uri, [string]$username, [string]$password) { $ftp = [System.Net.FtpWebRequest]::Create($uri) $ftp = [System.Net.FtpWebRequest]$ftp $ftp.Method = [System.Net.WebRequestMethods+Ftp]::DeleteFile $ftp.Credentials = new-object System.Net.NetworkCredential($username,$password) $rs = $ftp.GetResponse() $rs.Close() $rs.Dispose() } Function FtpUpload ([System.Uri]$uri, [string]$username, [string]$password, [string]$filePath) { $ftp = [System.Net.FtpWebRequest]::Create($uri) $ftp = [System.Net.FtpWebRequest]$ftp $ftp.Method = [System.Net.WebRequestMethods+Ftp]::UploadFile $ftp.Credentials = new-object System.Net.NetworkCredential($username,$password) $ftp.UseBinary = $true $ftp.UsePassive = $true $content = [System.IO.File]::ReadAllBytes($filePath) $ftp.ContentLength = $content.Length $rs = $ftp.GetRequestStream() $rs.Write($content, 0, $content.Length) $rs.Close() $rs.Dispose() } $myDir = Split-Path -Parent $MyInvocation.MyCommand.Path $updatesFileName = "MyApplicationUpdates.txt" $updatesPath = $myDir + "\" + $updatesFileName $installerFileName = "MyApplicationSetup.exe" $installerPath = $myDir + "\" + $installerFileName if (Test-Path $updatesPath) { Remove-Item $updatesPath } $releaseNotesLines = [IO.File]::ReadAllLines("ReleaseNotes.txt") $u = ";aiu;`n" $u += "`n" $u += "[My Application 3]`n" $u += "Name=My Application $RedGateReleaseNumber`n" $u += "ProductVersion=$RedGatePackageVersion`n" $u += "UpdatedApplicationVersions=>=3`n" $u += "URL=$httpDownloadsUrl/$installerFileName`n" $u += "Size="+(Get-Item $installerPath).Length+"`n" $u += "ServerFileName=$installerFileName`n" $u += "Flags=NoCache`n" $u += "FilePath=[APPDIR]MyApplication.exe`n" $u += "Version=$RedGatePackageVersion`n" $lineNumber = 0; foreach($line in $releaseNotesLines){ $descriptionNumber = "" if ($lineNumber -gt 0) { $descriptionNumber = $lineNumber } $u += "Description$descriptionNumber=$line`n" $lineNumber++ } $u += "AutoCloseApplication=[APPDIR]MyApplication.exe`n" $u | out-file $updatesPath -encoding ASCII FtpUpload (new-object System.Uri "$ftpDownloadsUrl/$updatesFileName") $ftpUserName $ftpPassword $updatesPath FtpUpload (new-object System.Uri "$ftpDownloadsUrl/$installerFileName") $ftpUserName $ftpPassword $installerPath
I hope this helps,
Justin
Justin Caldicott
Developer
Redgate Software Ltd
Developer
Redgate Software Ltd