Hit count is incorrect??
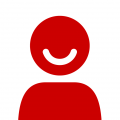
Hi,
I use ATNS Performance Profiler v7.4 to check an insertion sort algorithm.
I am sure that the line of outer loop, the line 28, should be hit 10,000 times, as the input array size is 10,000.
But I noticed that the hit count sometimes is 10,000, and sometimes is just a random number, like 9940, 9909, 9933, etc.
Any suggestion?
Thanks.
Software: Windows 7 sp1 64 bit, Visual Studio 2010 sp1, C#
Hardware: CPU Intel i5 760, Memory 12G DDR3

1. using System;
2. using System.Collections.Generic;
3. using System.Linq;
4. using System.Text;
5.
6. namespace ConsoleApplication1
7. {
8. class Program
9. {
10. static private int _countOuterloop;
11.
12. static void Main(string[] args)
13. {
14. int[] theArray = new int[10000];
15.
16. PrepareArray(ref theArray); // fills the array with random numbers
17.
18. InsertionSort(theArray);
19.
20. }
21.
22.
23. static void InsertionSort(int[] theArray)
24. {
25. _countOuterloop = 0;
26.
27. int lenth = theArray.Length;
28. for (int j = 1; j < lenth; j++) // The array size is 10,000, this line should be hit 10,000 times
29. {
30. int key = theArray[j];
31. int i = j - 1;
32. while (i >= 0 && theArray > key)
33. {
34. theArray[i + 1] = theArray;
35. i--;
36. }
37.
38. theArray[i + 1] = key;
39. _countOuterloop++;
40. }
41.
42. }
43.
44.
45.
46. static void PrepareArray(ref int[] theArray)
47. {
48. int length = theArray.Length;
49.
50. Random theRandom = new Random();
51.
52. for (int i = 0; i < length; i++)
53. {
54. theArray = theRandom.Next(-length, length);
55. }
56. }
57.
58.
59. }
60. }
I use ATNS Performance Profiler v7.4 to check an insertion sort algorithm.
I am sure that the line of outer loop, the line 28, should be hit 10,000 times, as the input array size is 10,000.
But I noticed that the hit count sometimes is 10,000, and sometimes is just a random number, like 9940, 9909, 9933, etc.
Any suggestion?
Thanks.
Software: Windows 7 sp1 64 bit, Visual Studio 2010 sp1, C#
Hardware: CPU Intel i5 760, Memory 12G DDR3

1. using System;
2. using System.Collections.Generic;
3. using System.Linq;
4. using System.Text;
5.
6. namespace ConsoleApplication1
7. {
8. class Program
9. {
10. static private int _countOuterloop;
11.
12. static void Main(string[] args)
13. {
14. int[] theArray = new int[10000];
15.
16. PrepareArray(ref theArray); // fills the array with random numbers
17.
18. InsertionSort(theArray);
19.
20. }
21.
22.
23. static void InsertionSort(int[] theArray)
24. {
25. _countOuterloop = 0;
26.
27. int lenth = theArray.Length;
28. for (int j = 1; j < lenth; j++) // The array size is 10,000, this line should be hit 10,000 times
29. {
30. int key = theArray[j];
31. int i = j - 1;
32. while (i >= 0 && theArray > key)
33. {
34. theArray[i + 1] = theArray;
35. i--;
36. }
37.
38. theArray[i + 1] = key;
39. _countOuterloop++;
40. }
41.
42. }
43.
44.
45.
46. static void PrepareArray(ref int[] theArray)
47. {
48. int length = theArray.Length;
49.
50. Random theRandom = new Random();
51.
52. for (int i = 0; i < length; i++)
53. {
54. theArray = theRandom.Next(-length, length);
55. }
56. }
57.
58.
59. }
60. }
Comments
Thanks for the example code. I tried running the profiler on it but I'm afraid I wasn't able to reproduce the issue. I wonder.. perhaps you had different parts of the timeline selected each time it gave a different hit count? Can you please try selecting the entire timeline and see if that gives 10000? The timeline can also get a bit out of sync (generally only by a few seconds) so it can help to select a slightly larger region if that's in use.
Jessica Ramos | Product Support Engineer | Redgate Software
Have you visited our Help Center?
Jessica Ramos | Product Support Engineer | Redgate Software
Have you visited our Help Center?