Options
Generating IDs greater than the current max
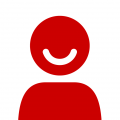
Hi There,
We have a PK column that is essentially an interger ID. We need to generate data for this table each day.
SDG seems to generate the same integers each time it runs, causing a PK violation.
Is there any way to make the integer start at the maximum current value of the column +1 ?
Many thanks!
We have a PK column that is essentially an interger ID. We need to generate data for this table each day.
SDG seems to generate the same integers each time it runs, causing a PK violation.
Is there any way to make the integer start at the maximum current value of the column +1 ?
Many thanks!
Comments
Typically in this case SQL Data Generator would just work because you would have set the column as an IDENTITY and SQL Data Generator would pick up at the next increment value.
However, if the column does not have the identity property set, you have to use the Python generator to query the table for the latest value and then start generating numbers from the next value. Here is an example:
This works mostly.
Its ok for normal ints, but when we have a BigInt ID Column, it fails:
Next ID would be 50000000510593201
So I changed it to use an int64:
rowCount=int(reader.GetInt64(0))
The correct values then appear on SDG, but they are Orange and have a yellow exclimation mark on the column.
When I try to run the generate, it says
Value '50000000510593201' is the wrong data type for column ID
How do I get it to insert BigInt Columns?
Thanks very much.