Execute sql packager package in a automated update program .
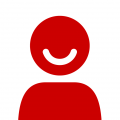
I have a windows service that runs on a DB server of a customer.
This service can download the sqlpackage.exe that sql packager creates.
I want to execute the package via my program passing in the correct command line parameters via c# and wait until it finishes..
Has anyone attempted something similar and where could I find an example....
This service can download the sqlpackage.exe that sql packager creates.
I want to execute the package via my program passing in the correct command line parameters via c# and wait until it finishes..
Has anyone attempted something similar and where could I find an example....
Comments
It's really easy to start a process in C#. Basically this:
You probably also want to plumb in some logic to wait for the process and capture the output from the package. You can use p.WaitForExit() to block your service until the package is finished and then check the p.ExitCode property to see what the numeric result was. In a service, nobody will see the output, though, so you have to hide the window and optionally send the package output somewhere else like a log file, etc. Maybe the latter is a bit complicated, but I had the source handy. Hope it helps.
Thanks