App not exiting after exception?
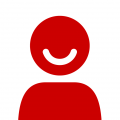
Something really strange seems to happen in this little commandline app: when the exception is thrown, the app does not terminate when processed with SA.
This is just a really simple app which should just do its job while providing feedback in the background on a separate thread. No big design, just a hack. I reduced the code to the minimum to repro the case.
Without SA the app exits as expected when the exception occurs. With SA it seems to terminate only the main thread.
This is just a really simple app which should just do its job while providing feedback in the background on a separate thread. No big design, just a hack. I reduced the code to the minimum to repro the case.
Without SA the app exits as expected when the exception occurs. With SA it seems to terminate only the main thread.
namespace test { public class Program { private Thread dummy = new Thread(new ThreadStart(DummyDisplay)); public static void Main(string[] args) { Program me = new Program(); me.dummy.Start(); throw new ArgumentException(); // continue program here } private static void DummyDisplay() { while(true) { // do some processing, and then sleep for a long time } } } }
Comments
If you are using SmartAssembly's error reporting feature, there is an option to "continue" on error. Did you use that option?
I'll have a look at the code on Monday try to work out what it's actually doing.
There are two types of threads in .NET; background threads will automatically get terminated when an application ends, whereas all foreground threads must exit before the application will exit.
Without SA the exception will reach to the JIT debugger which is the only thing that can end all of a processes threads instantly.
SA has to add the code to handle the exception otherwise it would fall to the JIT debugger and the user would get two error messages. The most that SA can do is tell the application to exit in every way possible. It could add some really nasty hacky code which went through and terminated all of the threads in the current process, but this wouldn't handle all of the tidying up (e.g. of unmanaged resources) of the other threads.
Only thing I can really suggest is to make the thread a background thread (thread.IsBackground = true), as usually you don't need a foreground thread.